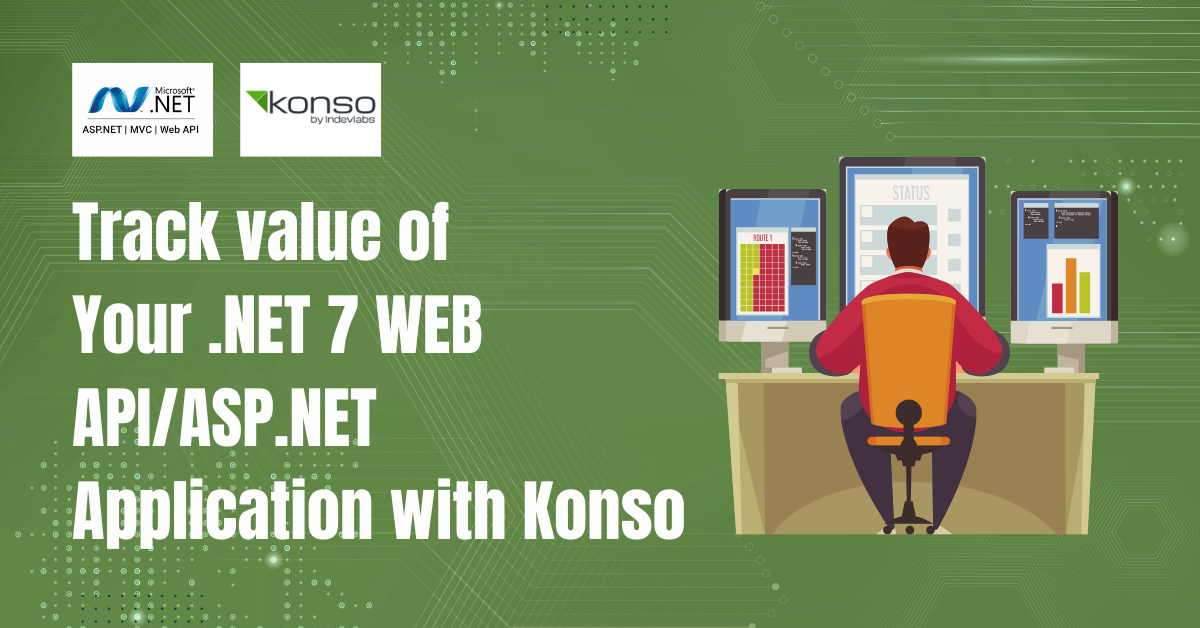
Applying Value Tracking for your .NET 7 application with Konso
When developing applications, the primary focus is often on catering to the needs of businesses. It is crucial for business owners to have a real-time understanding of how their ventures are performing. In the context of applications and digital products, it becomes essential to capture business events accurately. This enables product owners to assess the usage patterns within their applications, identifying which parts are utilized more frequently and which are used less.
When you deliver a new feature its interesting for you as a product owner how it is adopted by users, so you can make decision and set up a healthy feedback loop.
A user action, a transaction, or any other significant event that brings value counts.
Value tracking helps bridge the gap between development and business objectives by providing measurable data on the application's performance, adoption of new features, user behavior patterns, and overall user satisfaction. It plays a crucial role in enhancing the decision-making process and enables iterative improvements based on real-time feedback and usage analytics.
Konso as part of application observability feature offers you real-time analytics and reporting features to gain actionable insights from your captured event data.
In this post, I will demonstrate the process of adding value tracking into your .NET project using Konso. We will explore a practical example by implementing it on a simple "To Do" application, which can be found on GitHub
Konso Account
Create a Konso account by filling simple registration form
Create a new bucket
A bucket in Konso is like a container that holds a set of specific functions or features. It is a fundamental part of the Konso data structure and serves as a way to organize and group together related functionalities. Think of it as a collection of chosen tools or capabilities that are grouped together for a specific purpose.
Name - Name of a bucket e.g. "ToDo DEV", "ToDo PROD", I organize data by environment.
Description - Write a description for a bucket.
Install the library via NuGet or by manually referencing the assembly in your project
NuGet\Install-Package Konso.Clients.ValueTracking
Initialize the library with your API credentials and configuration settings
Add config to appsettings.json
:
"Konso": {
"ValueTracking": {
"Endpoint": "https://apis.konso.io",
"BucketId": "",
"ApiKey": "<bucket's access key>"
}
}
in startup.cs
:
builder.Services.AddKonsoValueTracking(options =>
{
options.Endpoint = builder.Configuration.GetValue("Konso:ValueTracking:Endpoint");
options.BucketId = builder.Configuration.GetValue("Konso:ValueTracking:BucketId");
options.ApiKey = builder.Configuration.GetValue("Konso:ValueTracking:ApiKey");
});
Now, when initial setup is ready we can deal with a business part:
Capturing business events
To begin capturing business events, it is essential to determine which events are considered valuable. In my scenario, I have identified three such events:
- Task Creation
- Task Update
- Task Delete
To facilitate this, I have created an enum called "ValueTrackingTypes" to define these significant events:
public enum ValueTrackingTypes
{
CreateTask = 1, UpdateTask = 2, DeleteTask = 3,
}
These eventIds, represented by the assigned numbers, will be utilized later when streaming events to Konso, allowing for the proper identification and tracking of these specific events.
In Konso, it is possible to map numeric IDs to captions, which helps to make them more user-friendly when used in charts and reports. This feature allows for the association of meaningful labels or descriptions with numeric identifiers, making it easier to interpret and present data visually. By mapping numeric IDs to captions, Konso enables a more intuitive and comprehensible representation of information in charts and reports.
Create a mapping for event IDs
Create a mapping for event IDs, in Konso, by navigating to Observability/Value Tracking/Mapping
id - The id of event in your system.
name - Name of event is should be presented on chart and reports.
description - Description of event.
value - Specify what value event brings e.g 1 point/euro/dollar.
These are mappings I've added:
Code level capturing
Then, we can actually define those events in code. For that I defines a KonsoModel
class for my razor pages derived from a PageModel
. This class extends base class with a TrackValue
Method:
public class KonsoModel : PageModel
{
internal readonly IValueTrackingClient _valueTrackingService;
public KonsoModel(IValueTrackingClient valueTrackingService)
{
_valueTrackingService = valueTrackingService;
}
internal async Task TrackValue(string user, ValueTrackingTypes type, double? value = null)
{
await _valueTrackingService.CreateAsync(new ValueTrackingCreateRequest()
{
IP = Request != null ? Request.HttpContext.Connection.RemoteIpAddress.ToString() : string.Empty,
EventId = (int)type,
User = user,
Value = value,
CorrelationId = Request.HttpContext.Session.Id,
Browser = Request.Headers\[HeaderNames.UserAgent\],
AppName = "todoapp",
Tags = new List() { Environment.GetEnvironmentVariable("ASPNETCORE\_ENVIRONMENT") }
});
}
}
Note: By default, the user property is hashed during processing, ensuring the anonymization of the user's data. Hashing is a technique that transforms the user's identifiable information into a unique, irreversible string of characters. This process protects the user's privacy by removing any direct link between their personal data and the hashed representation. It provides an added layer of security and confidentiality when handling user information within the system.
Run the app generate some events and explore them in the Konso.
You can explore and see the overview of your business events
In conclusion, implementing value tracking in your .NET 7 application with Konso can greatly enhance your understanding of how your application is performing and bring valuable insights into user behavior. By capturing and analyzing important business events, such as task creation, update, and deletion, you can make informed decisions and set up a healthy feedback loop. Mapping numeric IDs to captions in Konso also enables more user-friendly charts and reports, improving data visualization. Incorporating value tracking empowers product owners to optimize their applications, prioritize features, and ultimately deliver a more satisfying user experience. Embracing this approach will not only benefit your current project but also lay the foundation for data-driven decision-making in future endeavors.
No comments are allowed for this post